Xwa consists of two distributions:
- user distribution
- developer distribution
In both cases, no admin rights are required
Deployment process can be as simple as user opening an Office document. Then if installation/update has to be performed, it is automated from that point. That way, no system administrator is involved. Of course, installations can be also authorized with admin credentials prompt.
Xwa-User-distribution
User-distribution is a runtime package that is installed on user computer. It provides all functionality needed by solutions developed with Xmart Web Api.
Xwa-Developer-distribution
Developer-distribution consists of SDK and tools used for efficient development. It is intended to be used by VBA developers.
How can Xwa connect without connection strings?
Using tools provided with Xmart Web Api, it is possible to embed encrypted data directly into the Office file structure. Developer can decide where to keep encrypted values:
- in key vault service (hosted in cloud or company’s network)
- or within Office file.
Either way, no additional files or registry entries are produced. Connection strings are always encrypted and never exposed. In VBA code it is sufficient to use an enum (or any numeric value) – instead of strings that contain vulnerable data.
For more information, please see the Q&A Page.
Example (ported) code
The example below demonstrates how Xmart Web Api works with usual VBA code that uses ADO:
Enum MyConnections LocalDemo = 1 CloudDemo = 2 End Enum Sub XwaConnectionExample() Dim xwa As New XmartWebApiConnection ' creates xwa wrapper instance ' usually at some point connection string is exposed: ' -------------------------------------------------------------------------------------------------------------- ' Dim conn As New ADODB.Connection ' conn.ConnectionString = "Provider=SQLOLEDB;Server=.\SQLEXPRESS;Database=myDb;UID=myUsername;PWD=myPassword;" ' -------------------------------------------------------------------------------------------------------------- ' with xwa, there is no connection string, just enumeration that points to the data source If xwa.OpenConnection(CloudDemo, openTransaction:= false) Then Dim cmd As New ADODB.Command ' ado command With cmd .CommandText = "myUpdateProc" ' ado parameters .Parameters.Append .CreateParameter("@MyParam1", adInteger, adParamInputOutput, 0, 1) .Parameters.Append .CreateParameter("@MyParam2", adVarWChar, adParamInput, 128, "text") End With Dim rowsAffected As Long xwa.Execute cmd, rowsAffected ' executes data update cmd.CommandText = "mySelectProc" Dim rs As ADODB.Recordset Set rs = xwa.RefreshRecordset(cmd) ' selects data to ado recrodset CopyFromRecordSet rs, Worksheets("my worksheet").Range("A1") ' copies recordset to worksheet's range Set rs = Nothing Set cmd = Nothing End If Set xwa = Nothing End Sub
As you can see, it is very easy to update existing solutions. It can either be done manually or using tools that come with the developer distribution.
You don’t have to write code
With Xwa ORM tools it is possible to generate entire data access layer. Objects from database are mapped to classes and worksheets. You can also generate SQL objects that reflect worksheets. SQL syntax for reading and writing can also be generated.
This significantly speeds up the development process.
If you use Xwa tools for your development, you don’t have to know code used for database programming. Instead, you can focus on other parts of your Office application.
Publishing to end users and additional protection
When Office solution is ready to be published, Xwa tools can be used to automate that process.
Usually, publishing to end users involves these steps: code signing, time-stamping, a backup, uploading to distribution system etc.
It is also possible to setup custom events, in with you can fire your own macros or batch programs. Because of that, you can implement any non-typical publish behavior.
With Xwa, you can also add additional protection constraints. For example, any unprotection attempts or post-publish code changes will put all exported connections in an unusable state.
To sum it up
The usual flow of VBA development using Xmart Web Api:
- Generate VBA/SQL with ORM tools
- Develop your application
- Publish to end users
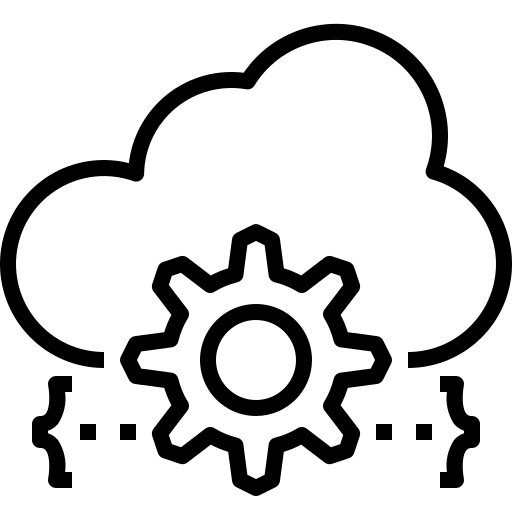